Singular queries
To understand your first query with GraphQL, we will start with something easy. Let's try to query a single customer:
query {
Customer (id: 100012) {
id
label
}
}
- First, we need to tell GraphQL that we will be querying data. this is done by the first
query
string. - Then we tell which type of entity we want to query and give the
id
of the Customer as a parameter.
To get this id, just open Stockpit, select the customer, and get this number from the URL displayed in your browser address bar
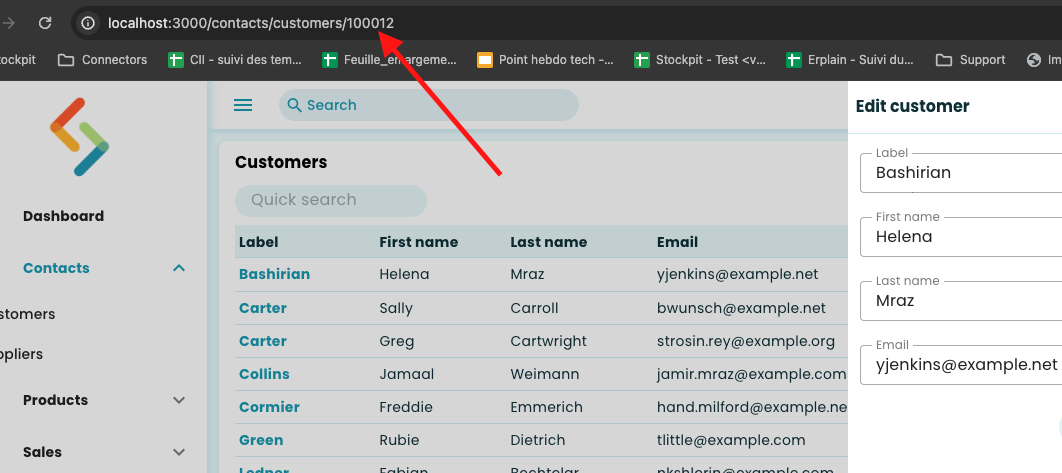
- We finally add the fields we need to query. GraphQL is very flexible, meaning that you can add or remove the fields you want to tailor the query to your needs.
id
andlabel
are common to most of the entities in Stockpit.
Here is the result of the query:
{
"data": {
"Customer": {
"id": 100003,
"label": "Bashirian"
}
}
}
Plural queries
If you want to query multiple customer at once. You can do this like this:
query {
Customers {
data {
id
label
}
}
}
Notice that the query Customer
became Customers
when we decided to return multiple results. Almost every query has two forms: a singular form (which only returns a single result) and a plural form (which returns multiple results). For ease of use, these queries are simply the singular and plural version of the same word (ex: Supplier
=> Suppliers
, Customer
=> Customers
, ... ) but if you need a reminder, you can find all the available queries in this documentation, on their respective entity's pages.
We will learn in the Pagination page how to add pagination to that query.
Singular sub-queries
Some fields requires sub-queries to other entities. For exemple, to get the address of a Supplier
, you need to do a sub-query like this:
query {
Supplier (id: 100343) {
id
label
address {
address_1
country
postal_code
city
}
}
}
address
is a sub-query to the Address entity.- Like any singular query, you can ask any field about the address that you need.
Plural sub-queries
Some sub-queries return multiple results at once, like Products
and Variants
for example. You need to call them like this:
query {
Product (id: 101342) {
id
label
variants {
data {
id
label
}
}
}
}
- The syntax of a plural sub-query is the same as a plural query.
- Pagination is available for sub-queries.
Sending multiple queries
GraphQL is great and one of its features is to allow you to send multiple queries at once.
query {
Customers {
data {
id
label
}
}
Products {
data {
id
label
}
}
}
The returned value will look like
{
"data": {
"Customers": {
"data": [
{
"id": 100000,
"label": "Bauch"
},
{
"id": 100001,
"label": "Glover"
}
]
},
"Products": {
"data": [
{
"id": 100000,
"label": "Extraordinary Pants"
},
{
"id": 100001,
"label": "Shiny Pants"
}
]
}
}
}